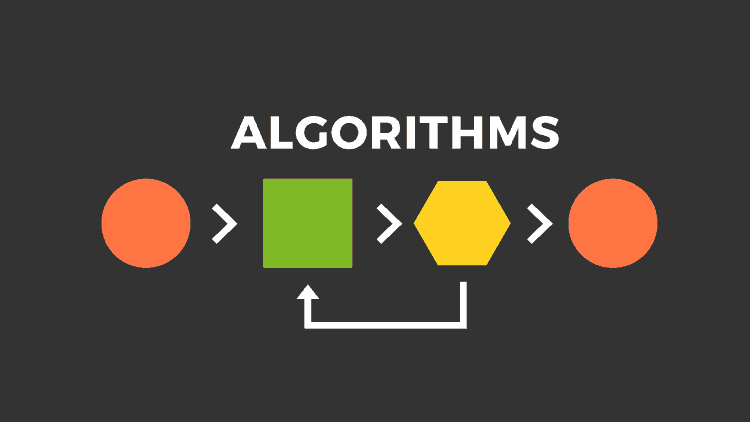
Demystifying Algorithms: A Beginner’s Guide to understand Algorithms (Avoid being a Bogo Sort developer)
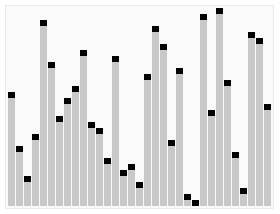
As a software engineer, when you are required to develop a logical feature or functionality, you will be developing an Algorithm, which may sound sofisticated and complex, and they can really be, but in reality an Algorithm is just a sequence of logical steps or instructions used for solving a specific problem.
For example, you are required to display the sales data in an alphabetical order by customer name, you will be implementing a sorting algorithm. The algorithm you choose will impact significantly the performance of your solution in resource usage (memory), complexity, speed, and other relevant factors that will be covered in this blog.
In most real world scenarios, a solution to a technical requirement will involve the development of multiple algorithms (sequence of instructions). What is important to know, is what algorithm should you develop to solve the technical requirement in an optimal way. This blog will share all you need to know about algorithms for real world tasks.
What are Algorithms?
Algorithms serve as the backbone of computer science and software engineering, providing step-by-step procedures for problem-solving and computation. For developers looking to embark on their software engineering journey, understanding the basics of algorithms is paramount. In this comprehensive blog, we’ll explore not only the fundamental algorithms but also how they are classified, their performance analysis, and real-world applications with easy-to-understand examples in Python.
In other words, algorithms:
- Are systematic procedures or rules used to solve problems or perform computations.
- They provide a clear set of instructions for carrying out a task or solving a specific problem.
- Algorithms are omnipresent in software development, from data sorting and searching to optimization and machine learning.
Importance of Understanding Algorithms
Imagine that you are requested to sort all the users that bought a concert ticket alphabetically by their name and display them within the web application, if you developed a bad algorithm you could overload the browser or server memory (depending if it was done in the frontend or backend, this will be discussed in further blogs about frontend and backend responsibilities) and this implementation made the application crash when sorting the users by their name. That would not be good as a software developer and this is why algorithms matter.
Therefore, the importance of understanding algorithms are the following:
- Efficient algorithms lead to optimized performance and faster execution of software applications.
- They enable engineers to tackle complex problems with precision and elegance.
- Understanding algorithms cultivates critical thinking and problem-solving skills, essential for software development.
- There is no need to invent something new, when there are algorithms that can solve a problem efficiently.
Before developing an algorithm, you must evaluate the context of the feature to develop and identify the following details:
- Feature Domain: does it involve a search, sort, graph or string problem?
- Data Sources: does the initial data structures that support your algorithm, are in a hash map, list or working with a queue? Probably you will need to mutate the initial data structures depending on the algorithm you need to develop.
- Resource Limitations: are you performing the algorithm in the users browser, cloud servers, or in remote services?
Once you idetify these details, you can develop the algorithm that best fits those requirements.
For each Algorithm, take into consideration:
- Problem Constraints
- Best suited data structure for the algorithm (tree, linked lists, lists, queue or stacks)
- Data types
- Algorithm objective (sort, search, explore)
- Input size (some algos outperform others based on the input size)
- Working with balanced or unbalanced trees
- Repeated values (some algos does not work well with repeated values)
- Space and Time complexity of the algorithm
- Problem Constraints: Understanding the constraints of the problem is crucial. Factors such as input size, data type, and specific requirements can influence the choice of algorithm. For example, if the input is large, efficiency becomes paramount, while if the input is small or specific conditions apply, a simpler algorithm may suffice.
- Time Complexity: Evaluating the time complexity of candidate algorithms relative to the problem’s requirements is essential. Algorithms with lower time complexities are generally preferred, especially for larger inputs or when fast execution is critical.
- Space Complexity: Similar to time complexity, the space complexity of algorithms should be considered. Some algorithms may have lower time complexities but higher space complexities, and vice versa. Choosing an algorithm with an appropriate balance between time and space complexity is often necessary.
- Input Characteristics: Understanding the characteristics of the input data can guide algorithm selection. For example, if the data is already partially sorted, certain sorting algorithms like Insertion Sort or Bubble Sort may perform better than others.
- Stability and Stability: Consider whether the algorithm needs to maintain the order of equal elements (stability) or not. Certain applications may require stable sorting or traversal algorithms, while others may not.
Classification of Algorithms
There are several ways to classify algorithms, each offering a distinct perspective on their characteristics. Here are some prominent categories:
If you would like to run these algorithms, copy the code blocks in a google collab notebook.
import random
import matplotlib.pyplot as plt
import heapq
def generate_random_array(size):
return [random.randint(1, 100) for _ in range(size)]
def select_random_value(arr):
return random.choice(arr)
# Generate a dynamic array of size 10
dynamic_array = generate_random_array(10)
# Select a random value from the array to search for
target = select_random_value(dynamic_array)
print("Random value selected for search:", target)
print(dynamic_array)
By Functionality (Search, Sort and Traversal)
Search Algorithms: Designed to find specific data within a dataset. (e.g., Linear Search, Binary Search)
Linear Search
Linear search, also known as sequential search, is the simplest search algorithm. It sequentially checks each element of the collection until it finds the target element or reaches the end of the collection. Linear search has a time complexity of O(n), where n is the number of elements in the collection and has constant space complexity O(1) as the memory required does not increase based on the input size.
def linear_search(arr, target):
steps = []
for i in range(len(arr)):
steps.append(i)
if arr[i] == target:
return True, steps
return False, steps
def visualize_linear_search(arr, target):
found, steps = linear_search(arr, target)
plt.plot(steps, [arr[idx] for idx in steps], marker='o', linestyle='-', color='b')
plt.xlabel('Index')
plt.ylabel('Value')
plt.title('Linear Search Visualization')
if found:
plt.annotate(f'Target found at index {steps[-1]} after {len(steps)} steps', xy=(steps[-1], arr[steps[-1]]), xytext=(steps[-1], arr[steps[-1]] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
else:
plt.annotate('Target not found', xy=(len(arr)-1, arr[-1]), xytext=(len(arr)-1, arr[-1] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
# Example usage with dynamic array
visualize_linear_search(dynamic_array, target)
Binary Search
Binary search is a more efficient search algorithm but requires that the collection be sorted beforehand. It works by repeatedly dividing the search interval in half until the target element is found. Binary search has a time complexity of O(log n), making it significantly faster than linear search for large collections.
def binary_search(arr, target):
low = 0
high = len(arr) - 1
steps = []
while low <= high:
mid = (low + high) // 2
steps.append(mid)
if arr[mid] == target:
return True, steps
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return False, steps
def visualize_binary_search(arr, target):
found, steps = binary_search(arr, target)
plt.plot(steps, [arr[idx] for idx in steps], marker='o', linestyle='-', color='b')
plt.xlabel('Index')
plt.ylabel('Value')
plt.title('Binary Search Visualization')
if found:
plt.annotate(f'Target found at index {steps[-1]}, after {len(steps)} steps', xy=(steps[-1], arr[steps[-1]]), xytext=(steps[-1], arr[steps[-1]] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
else:
plt.annotate('Target not found', xy=(len(arr)-1, arr[-1]), xytext=(len(arr)-1, arr[-1] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
# Example usage with dynamic array
visualize_binary_search(dynamic_array, target)
Depth-First Search (DFS)
Depth-First Search is a graph traversal algorithm that explores as far as possible along each branch before backtracking. It is often used to traverse or search through tree or graph data structures. DFS can be implemented recursively or iteratively using a stack data structure.
def dfs(arr, target):
visited = set()
steps = []
def recursive_dfs(node):
if node in visited:
return False
visited.add(node)
steps.append(node)
if arr[node] == target:
return True
for neighbor in [2*node + 1, 2*node + 2]:
if neighbor < len(arr):
if recursive_dfs(neighbor):
return True
return False
found = recursive_dfs(0) # Start with the root node
return found, steps
def visualize_dfs(arr, target):
found, steps = dfs(arr, target)
plt.plot(steps, [arr[idx] for idx in steps], marker='o', linestyle='-', color='b')
plt.xlabel('Index')
plt.ylabel('Value')
plt.title('DFS Visualization')
if found:
plt.annotate(f'Target found at index {steps[-1]} after {len(steps)} steps', xy=(steps[-1], arr[steps[-1]]), xytext=(steps[-1], arr[steps[-1]] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
else:
plt.annotate('Target not found', xy=(len(arr)-1, arr[-1]), xytext=(len(arr)-1, arr[-1] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
# Example usage with dynamic array
visualize_dfs(dynamic_array, target)
Breadth-First Search (BFS)
Breadth-First Search is another graph traversal algorithm that systematically explores all neighbor nodes at the present depth before moving on to the nodes at the next depth level. BFS is often used to find the shortest path in unweighted graphs or to explore the structure of a graph.
def bfs(arr, target):
queue = deque()
visited = set()
steps = []
queue.append(0) # Start with the root node
while queue:
node = queue.popleft()
if node in visited:
continue
visited.add(node)
steps.append(node)
if arr[node] == target:
return True, steps
for neighbor in [2*node + 1, 2*node + 2]:
if neighbor < len(arr):
queue.append(neighbor)
return False, steps
def visualize_bfs(arr, target):
found, steps = bfs(arr, target)
plt.plot(steps, [arr[idx] for idx in steps], marker='o', linestyle='-', color='b')
plt.xlabel('Index')
plt.ylabel('Value')
plt.title('BFS Visualization')
if found:
plt.annotate(f'Target found at index {steps[-1]} after {len(steps)} steps', xy=(steps[-1], arr[steps[-1]]), xytext=(steps[-1], arr[steps[-1]] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
else:
plt.annotate('Target not found', xy=(len(arr)-1, arr[-1]), xytext=(len(arr)-1, arr[-1] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
# Example usage with dynamic array
visualize_bfs(dynamic_array, target)
A Search Algorithm*
A* (pronounced “A-star”) is a popular pathfinding algorithm used in artificial intelligence and robotics. It efficiently finds the shortest path between two nodes in a graph, taking into account both the cost to reach each node and a heuristic function that estimates the cost to reach the goal from that node.
def astar(arr, target):
heap = [(0, 0)] # (cost, node)
heapq.heapify(heap)
visited = set()
steps = []
while heap:
cost, node = heapq.heappop(heap)
if node in visited:
continue
visited.add(node)
steps.append(node)
if arr[node] == target:
return True, steps
for neighbor in [2*node + 1, 2*node + 2]:
if neighbor < len(arr):
heapq.heappush(heap, (abs(arr[neighbor] - target), neighbor))
return False, steps
def visualize_astar(arr, target):
found, steps = astar(arr, target)
plt.plot(steps, [arr[idx] for idx in steps], marker='o', linestyle='-', color='b')
plt.xlabel('Index')
plt.ylabel('Value')
plt.title('A* Search Visualization')
if found:
plt.annotate(f'Target found at index {steps[-1]} after {len(steps)} steps', xy=(steps[-1], arr[steps[-1]]), xytext=(steps[-1], arr[steps[-1]] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
else:
plt.annotate('Target not found', xy=(len(arr)-1, arr[-1]), xytext=(len(arr)-1, arr[-1] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
# Example usage with dynamic array
visualize_astar(dynamic_array, target)
Uniform Cost Search (UCS)
Uniform Cost Search is a variant of Dijkstra’s algorithm that finds the shortest path in a weighted graph. Unlike A*, which uses a heuristic function, UCS considers the actual cost of reaching each node from the start node and explores nodes in increasing order of cost.
def ucs(arr, target):
heap = [(0, 0)] # (cost, node)
heapq.heapify(heap)
visited = set()
steps = []
while heap:
cost, node = heapq.heappop(heap)
if node in visited:
continue
visited.add(node)
steps.append(node)
if arr[node] == target:
return True, steps
for neighbor in [2*node + 1, 2*node + 2]:
if neighbor < len(arr):
heapq.heappush(heap, (cost + 1, neighbor))
return False, steps
def visualize_ucs(arr, target):
found, steps = ucs(arr, target)
plt.plot(steps, [arr[idx] for idx in steps], marker='o', linestyle='-', color='b')
plt.xlabel('Index')
plt.ylabel('Value')
plt.title('Uniform Cost Search Visualization')
if found:
plt.annotate(f'Target found at index {steps[-1]} after {len(steps)} steps', xy=(steps[-1], arr[steps[-1]]), xytext=(steps[-1], arr[steps[-1]] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
else:
plt.annotate('Target not found', xy=(len(arr)-1, arr[-1]), xytext=(len(arr)-1, arr[-1] + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
# Example usage with dynamic array
visualize_ucs(dynamic_array, target)
Binary Search Tree (BST) Search
Binary Search Tree is a data structure that allows for efficient searching, insertion, and deletion of elements. In a BST, each node has at most two children, with the left child containing values less than the node and the right child containing values greater than the node. Searching in a BST has an average time complexity of O(log n), making it efficient for sorted data.
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return TreeNode(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
def inorder_traversal(root, steps):
if root:
inorder_traversal(root.left, steps)
steps.append(steps[-1] + 1 if steps else 1)
inorder_traversal(root.right, steps)
def bst_search(root, target):
steps = []
while root:
steps.append(steps[-1] + 1 if steps else 1)
if root.value == target:
return True, steps
elif root.value < target:
root = root.right
else:
root = root.left
return False, steps
def visualize_bst(arr, target):
root = None
for value in arr:
root = insert(root, value)
steps = [0]
inorder_traversal(root, steps)
found, steps = bst_search(root, target)
plt.plot(steps, [target] * len(steps), marker='o', linestyle='-', color='b')
plt.xlabel('Total Steps')
plt.ylabel('Value')
plt.title('Binary Search Tree Visualization')
if found:
plt.annotate(f'Target found after {steps[-1]} steps', xy=(steps[-1], target), xytext=(steps[-1], target + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
else:
plt.annotate('Target not found', xy=(steps[-1], target), xytext=(steps[-1], target + 5),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
# Example usage with dynamic array
visualize_bst(dynamic_array, target)
Sorting Algorithms
Sort algorithms: designed to organize data in a particular order.
Bubble Sort Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. It iterates through the list multiple times until no more swaps are needed, indicating that the list is sorted. Despite its simplicity, Bubble Sort is not efficient for large lists due to its time complexity of O(n^2). In terms of space complexity, Bubble Sort is an in-place sorting algorithm, meaning it requires only a constant amount of extra space O(1) as it sorts the list in situ.
def bubble_sort(arr):
n = len(arr)
steps = []
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
steps.append(list(arr))
return arr, steps
# Example usage with dynamic array
sorted_array, steps = bubble_sort(dynamic_array)
Selection Sort
Selection Sort divides the input list into two sublists: a sorted sublist and an unsorted sublist. It repeatedly selects the smallest (or largest) element from the unsorted sublist and moves it to the end of the sorted sublist. Selection Sort proceeds by finding the minimum element from the unsorted portion and swapping it with the first unsorted element, effectively growing the sorted sublist. While simple to implement, Selection Sort’s time complexity of O(n^2) makes it inefficient for large datasets. Like Bubble Sort, Selection Sort is an in-place algorithm with constant space complexity O(1).
def selection_sort(arr):
n = len(arr)
steps = []
for i in range(n):
min_idx = i
for j in range(i+1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
steps.append(list(arr))
return arr, steps
# Example usage with dynamic array
sorted_array, steps = selection_sort(dynamic_array)
Insertion Sort
Insertion Sort builds the final sorted array one item at a time by repeatedly taking the next item from the input data and inserting it into its correct position in the sorted part of the array. It works by iteratively consuming one input element each repetition and growing a sorted output list. Insertion Sort has an average and worst-case time complexity of O(n^2) but performs efficiently for small datasets or nearly sorted arrays. Similar to Bubble Sort and Selection Sort, Insertion Sort is an in-place sorting algorithm with constant space complexity O(1).
def insertion_sort(arr):
n = len(arr)
steps = []
for i in range(1, n):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
steps.append(list(arr))
return arr, steps
# Example usage with dynamic array
sorted_array, steps = insertion_sort(dynamic_array)
Merge Sort
Merge Sort is a divide-and-conquer algorithm that divides the input array into two halves, sorts each half separately, and then merges them back together in sorted order. It is known for its stable sorting and consistent O(n log n) time complexity. Unlike the previous sorting algorithms, Merge Sort requires additional space proportional to the size of the input array for temporary storage during the merging phase. Despite the additional space complexity of O(n), Merge Sort’s efficient performance makes it a popular choice for sorting large datasets.
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
left_half = arr[:mid]
right_half = arr[mid:]
merge_sort(left_half)
merge_sort(right_half)
i = j = k = 0
while i < len(left_half) and j < len(right_half):
if left_half[i] < right_half[j]:
arr[k] = left_half[i]
i += 1
else:
arr[k] = right_half[j]
j += 1
k += 1
while i < len(left_half):
arr[k] = left_half[i]
i += 1
k += 1
while j < len(right_half):
arr[k] = right_half[j]
j += 1
k += 1
return arr
# Example usage with dynamic array
sorted_array = merge_sort(dynamic_array)
Quick Sort
Quick Sort is another divide-and-conquer algorithm that selects a “pivot” element from the array and partitions the other elements into two sub-arrays according to whether they are less than or greater than the pivot. The sub-arrays are then sorted recursively. Quick Sort has an average time complexity of O(n log n) but can degrade to O(n^2) in the worst case, particularly when the pivot selection is poor. Despite its worst-case behavior, Quick Sort is often preferred for its average-case efficiency and in-place sorting, requiring only logarithmic extra space O(log n) for the recursion stack.
# Quick Sort
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
Traversal Algorithms
Traversal Algorithms are developed to s to explore various types of graphs and trees, enabling analysis of their structures and relationships by visiting every element in a data structure.
graph = {
'A': {'B', 'C'},
'B': {'A', 'D', 'E'},
'C': {'A', 'F'},
'D': {'B'},
'E': {'B', 'F'},
'F': {'C', 'E'}
}
start_vertex = 'A'
Depth-First Search (DFS)
Depth-First Search explores as far as possible along each branch before backtracking. It traverses a graph or tree data structure in a depthward motion and can be implemented recursively or iteratively using a stack. DFS is often used to detect cycles in graphs, explore connected components, or search for paths between nodes. Its time complexity is O(V + E), where V is the number of vertices and E is the number of edges in the graph, and its space complexity is O(V) for the recursion stack in the recursive implementation or for the stack data structure in the iterative implementation.
def dfs(graph, start):
visited = set()
stack = [start]
traversal = []
while stack:
vertex = stack.pop()
if vertex not in visited:
visited.add(vertex)
traversal.append(vertex)
stack.extend(graph[vertex] - visited)
return traversal
traversal_order = dfs(graph, start_vertex)
print("DFS Traversal Order:", traversal_order)
Breadth-First Search (BFS)
Breadth-First Search systematically explores all neighbor nodes at the present depth before moving on to the nodes at the next depth level. It traverses a graph or tree data structure in a level-order motion and can be implemented using a queue. BFS is often used to find the shortest path in unweighted graphs or to explore the structure of a graph. Its time complexity is O(V + E), where V is the number of vertices and E is the number of edges in the graph, and its space complexity is O(V) for the queue data structure.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
traversal = []
while queue:
vertex = queue.popleft()
if vertex not in visited:
visited.add(vertex)
traversal.append(vertex)
queue.extend(graph[vertex] - visited)
return traversal
# Example usage with dynamic array
traversal_order = bfs(graph, start_vertex)
print("BFS Traversal Order:", traversal_order)
Inorder Traversal
Inorder Traversal is a type of depth-first traversal specifically for binary trees. It recursively traverses the left subtree, visits the root node, and then recursively traverses the right subtree. In binary search trees, inorder traversal visits nodes in ascending order. Its time complexity is O(n), where n is the number of nodes in the binary tree, and its space complexity is O(n) for the recursion stack due to the depth of the tree.
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def inorder_traversal(root):
traversal = []
def recursive_inorder(node):
if node:
recursive_inorder(node.left)
traversal.append(node.value)
recursive_inorder(node.right)
recursive_inorder(root)
return traversal
# Example usage with dynamic array
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
inorder_order = inorder_traversal(root)
print("Inorder Traversal Order:", inorder_order)
By Design Paradigm:
- Divide and Conquer: Break down the problem into smaller, independent sub-problems (e.g., Merge Sort, Quick Sort)
- Dynamic Programming: Solve overlapping sub-problems efficiently by storing solutions (e.g., Fibonacci Sequence)
- Greedy Algorithms: Make the best immediate choice at each step, though not always resulting in the optimal solution (e.g., Fractional Knapsack Problem)
- Backtracking: explore all possible solutions by traversing a decision tree recursively. They backtrack when they reach an invalid solution and try alternative paths. Examples include the N-Queens problem and Sudoku solvers.
- Brute-Force: Simple approach that tries every possible solution (often inefficient for large datasets)
- Heuristics: Employs approximate methods to find good (but not guaranteed optimal) solutions
By Time and Space Complexity:
- Time Complexity: Analyzes the time an algorithm takes to execute based on the input size (e.g., Big O notation)
- O(n): Linear time (increases proportionally with input size)
- O(log n): Logarithmic time (increases slower than linear)
- Space Complexity: Measures the amount of extra memory an algorithm needs to run (also analyzed using Big O notation)
Understanding these classification schemes empowers you to:
- Choose the appropriate algorithm: Select the one that best suits the problem’s nature and data size.
- Analyze algorithm performance: Predict how the algorithm’s speed and memory usage scale with increasing input.
- Compare different algorithms: Evaluate the trade-offs between efficiency and optimality.
Remember, these categories often overlap. A single algorithm can belong to multiple classifications based on its properties. As you delve deeper into the world of algorithms, you’ll encounter more nuanced classification techniques that provide a comprehensive understanding of their strengths and weaknesses.
Divide and Conquer: powerful development paradigm for your developer career
In most real world scenarios, when you are requested to develop a feature, you will have to divide the requirement into multiple sub-problems, develop different algorithms for each sub-problem and then implement a “parent” algorithm that will join all the sub-problems algorithm to meet the feature requirements. As its name suggest, Divide and Conquer, is a development paradigm where you divide a requirement as a set of different problems that are required to be solved in order to conquer the final solution. When you feel stuck solving a problem, try dividing into sub-problems, conquer them independently and lastly, combine the solutions. Divide and Conquer algorithms can significantly improve efficiency and reduce redundant computations.
Remember:
- Divide:
- Break the problem into smaller, more manageable sub-problems. This step involves partitioning the input data into two or more smaller subsets. The sub-problems should ideally be similar in nature to the original problem but smaller in size.
- Conquer:
- Solve the sub-problems recursively. Each sub-problem is solved independently using the same algorithm until they are simple enough to be solved directly without further subdivision. This step often involves applying the same algorithm recursively to each sub-problem.
- Combine:
- Merge the solutions of the sub-problems to obtain the solution to the original problem. The solutions obtained from the sub-problems are combined or integrated to produce the final solution to the original problem.
Examples of Divide and Conquer Algorithms:
- Merge Sort:
- Merge Sort is a classic example of a Divide and Conquer algorithm used for sorting arrays or lists. It works by dividing the array into two halves, recursively sorting each half, and then merging the sorted halves to produce the final sorted array. Merge Sort has a time complexity of O(n log n) and is widely used for its efficiency and stability.
- Binary Search:
- Binary Search is another example of a Divide and Conquer algorithm used for searching elements in a sorted array or list. It works by dividing the array into halves and repeatedly narrowing down the search space by comparing the target element with the middle element of the array. Binary Search has a time complexity of O(log n) and is much more efficient than linear search for large datasets.
- Closest Pair of Points:
- This algorithm finds the closest pair of points in a set of points on a plane. It divides the set into two halves, recursively finds the closest pairs in each half, and then combines the solutions. This problem is often used in computational geometry and has applications in various fields such as computer graphics and geographic information systems.
Divide and Conquer algorithms are versatile and powerful tools for solving a wide range of computational problems efficiently. By breaking down complex problems into smaller, more manageable parts, Divide and Conquer algorithms enable us to tackle challenging problems with ease and elegance.
Leave a Reply